Introducing Types and Type Systems
Plan
-
Types and Type Systems
-
Typing rules for a simple language
-
Type checker for a simple language
-
Adding environments
-
Monomorphic Type Systems
-
Polymorphic Type Systems (next week)
Types and Type Systems
What kind of value results from evaluating these expressions?
-
n + 1
-
"hello" ^ "world"
-
(fn n ⇒ n * (n - 1))
-
if x < 0 then 1 else 0
Questions type systems can answer:
-
What kind of value does it evaluate to (if it terminates)?
-
What is the contract of the function (!)
-
Does this program contain certain kinds of errors?
-
Who has the rights to look at it?
-
Is the number miles or millimeters?
Questions type systems generally cannot answer:
-
Will my program contain a division by zero?
-
Will my program contain an array bounds error?
-
Will my program take
car
of'()
? -
Will my program terminate?
Static vs. Dynamic Checking
Most languages use a combination of static and dynamic checks:
Static:
-
input independent
-
efficient at run-time
-
approximate
Dynamic:
-
depends on input
-
run-time overhead
-
precise
What is a type?
-
As a working definition, a set of values
-
As a precise definition, a classifier for terms!!
Notea computation can have a type even if it never produces a value!
What is a type system?
-
As a working definition, an algorithm/interpreter/compiler that accepts or rejects programs.
-
As a precise definition, a judgement and inference rules that prove that an expression is well-typed.
Source of new language ideas for next 20 years
Needed if you want to understand advanced designs (or create your own).
Type System and Type Checker for a simple language
Q: What context do we need to evaluate an expression?
Q: Do we need all of the same information to determine the type of an expression?
-
If not, then what do we need?
Simple language
datatype bop = PLUS | MINUS | TIMES | DIVIDE
datatype cop = EQ | NE | LT | LE | GT | GE
datatype exp = E_Lit of int
| E_BinOp of bop * exp * exp
| E_CmpOp of cop * exp * exp
| E_IF of exp * exp * exp
| VAR of name
| SET of name * exp
| APPLY of name * exp list
datatype ty = T_INT | T_BOOL
type fun_ty = ty list * ty
Want to reject some expressions
Can’t add an integer and a boolean:
3 + (3 < 99)
let x = 3 in x + (x < 99)
Can’t compare an integer and a boolean
3 < (3 = 99)
let z = 3 = 99 in 3 < z
Type System and Type Checker
A type system specified with a judgement and inference rules:
-
Judgement form: \(\Gamma_\rho;\Gamma_\phi \vdash e : \tau\)
A type checker is code:
-
Signature:
val tychk: exp * ty env * (ty list * ty) env → ty
-
What are the possible behaviors of
tc
?
Develop inference rules and code for simple language.
Notes:
-
Inference rules only specify when an expression is well-typed, but type checking code needs to handle all of the different ways an expression can be ill-typed.
-
When a meta-variable appears more than once in an inference rule, it means that all occurrences are the same. In type checking code, need to explicitly check that types are equal.
Lecture Board Images
Type Systems: Motivations and Terminology
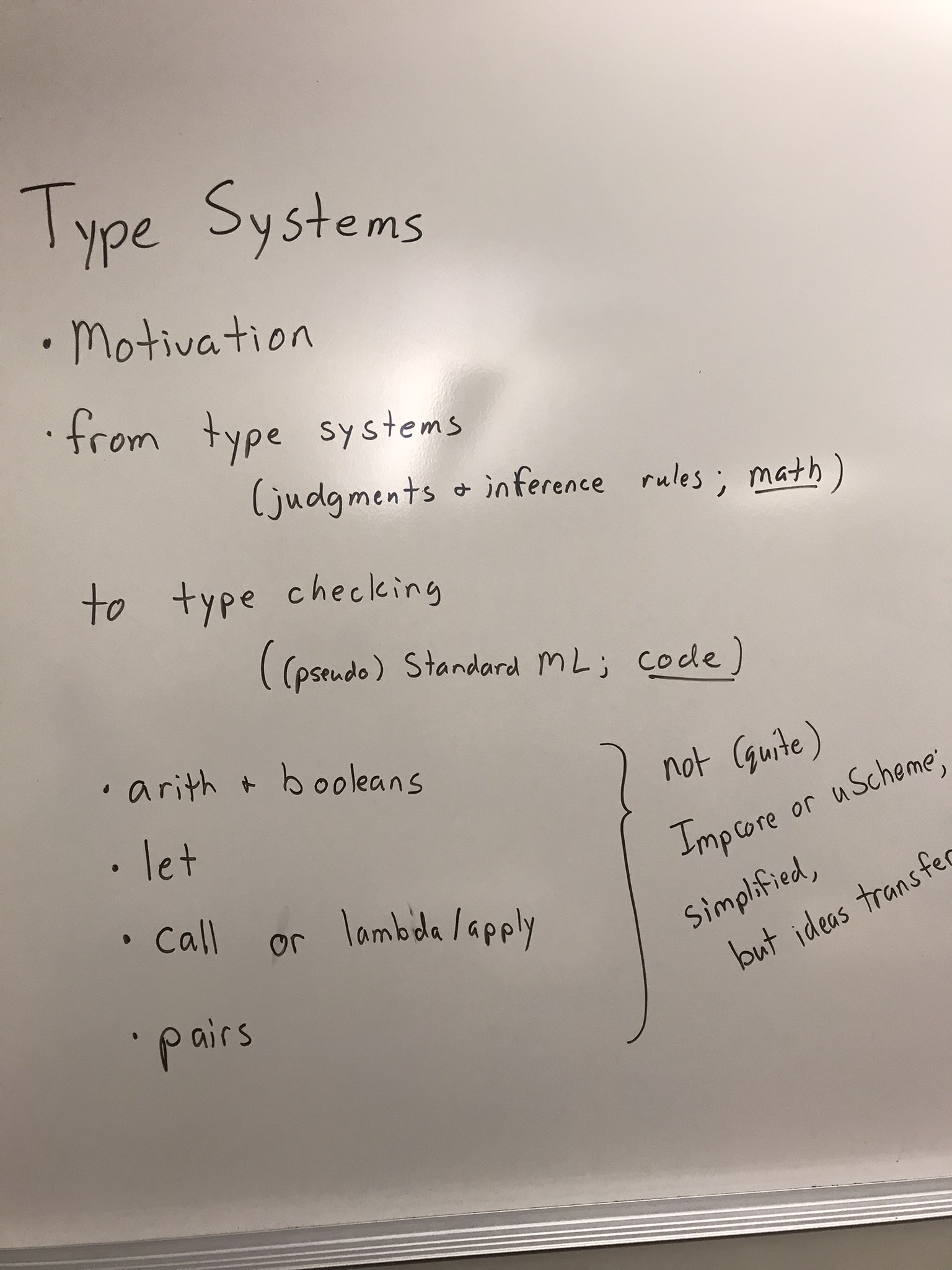
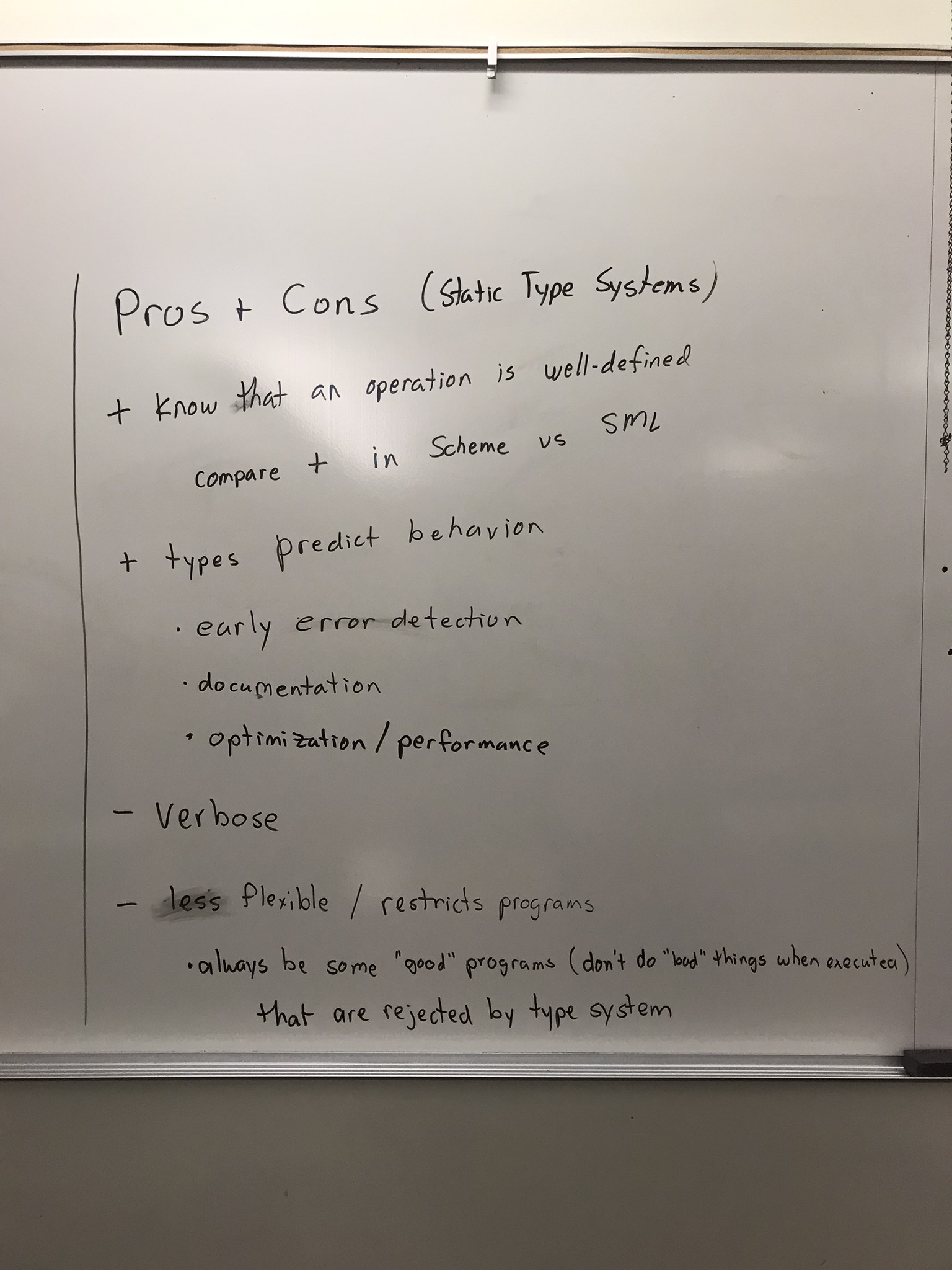
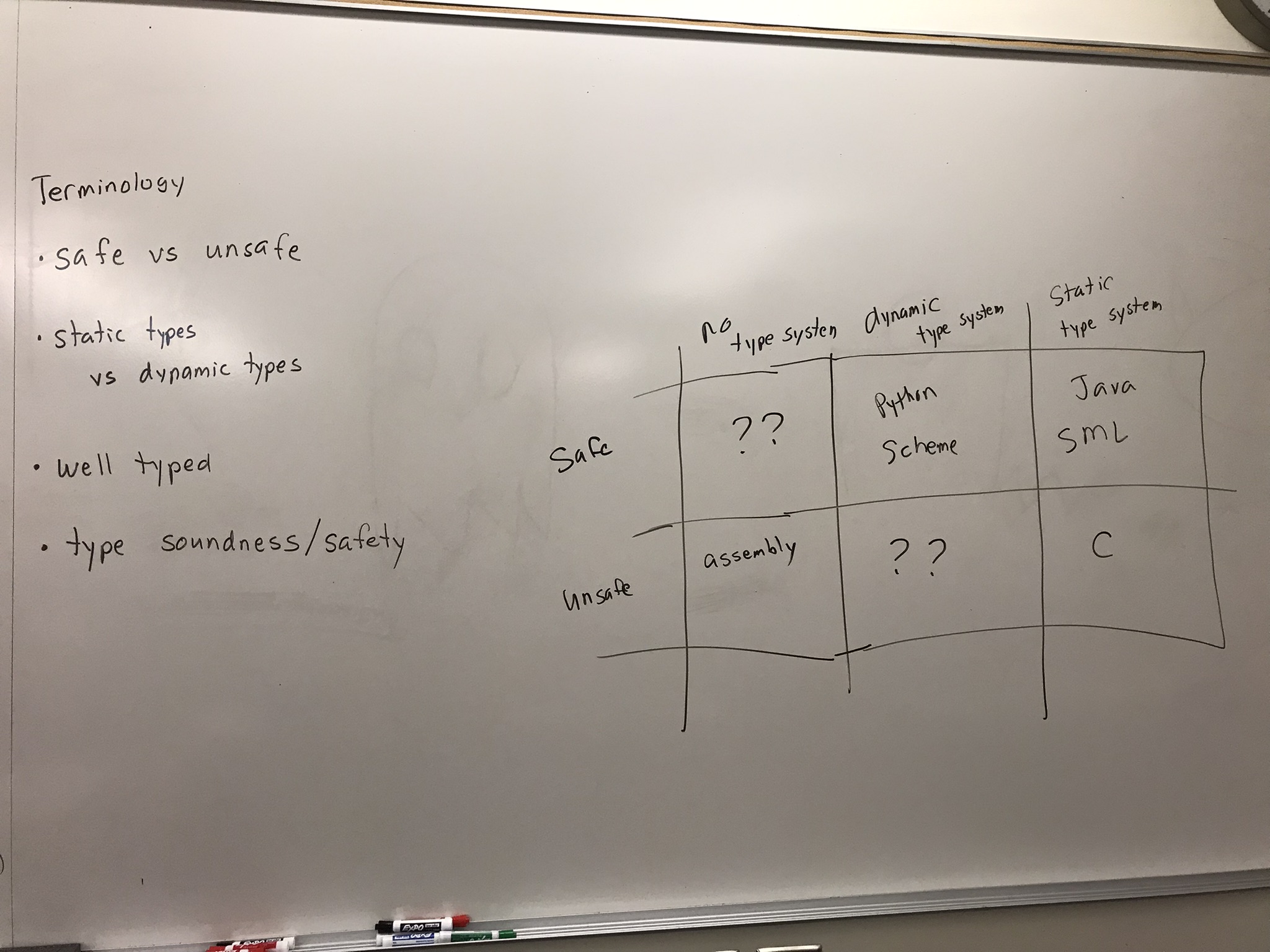
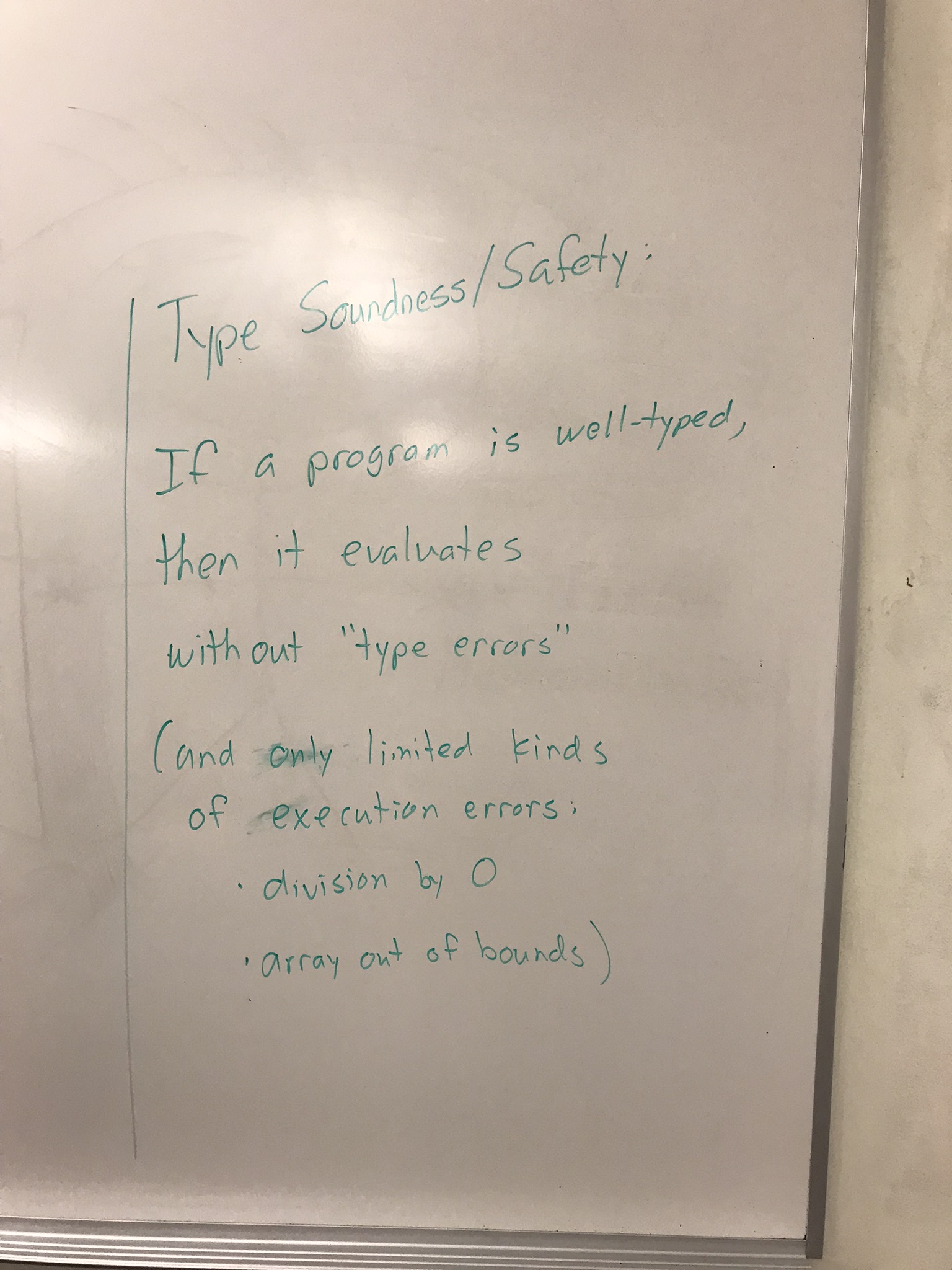
Micro Language
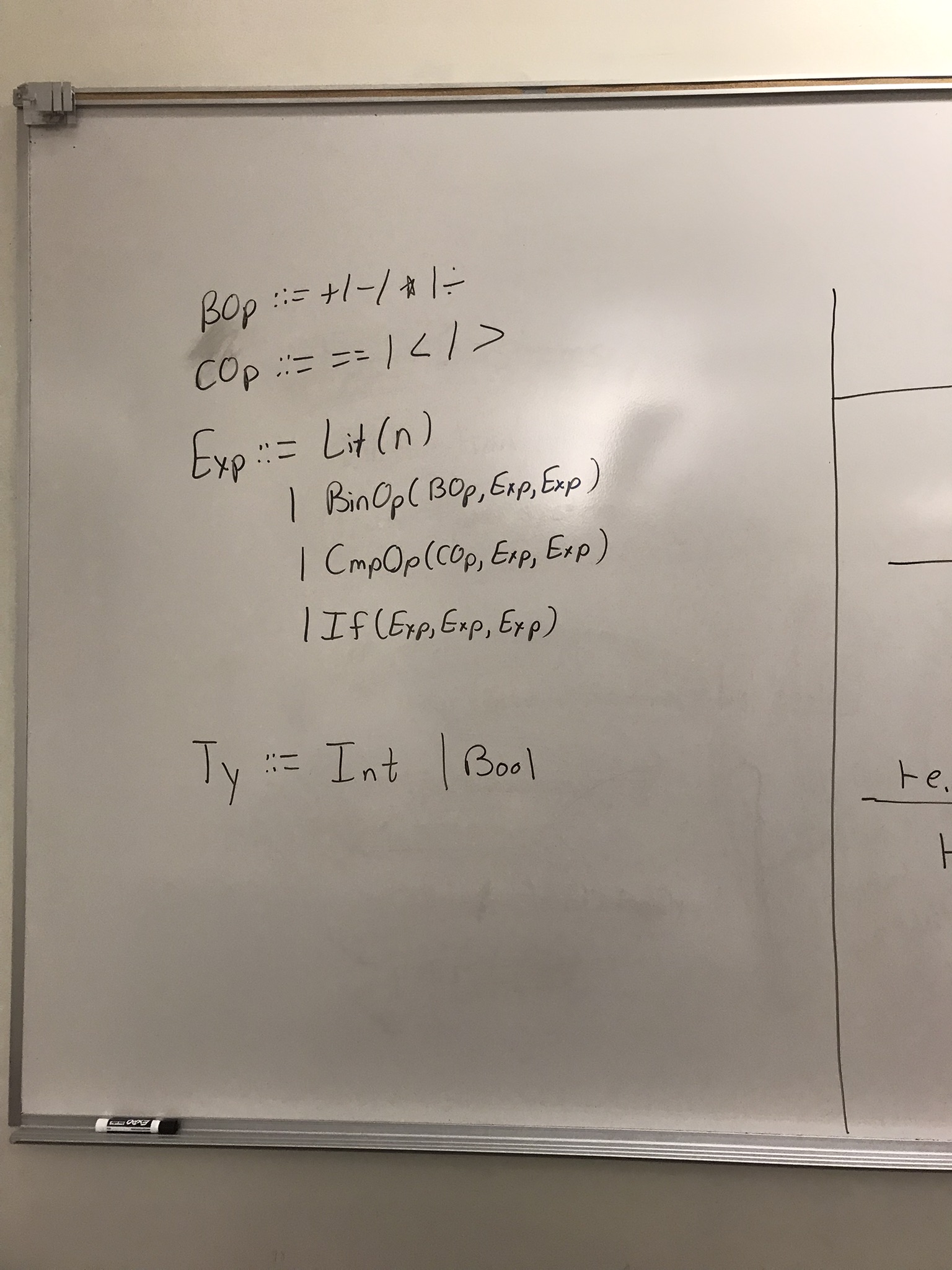
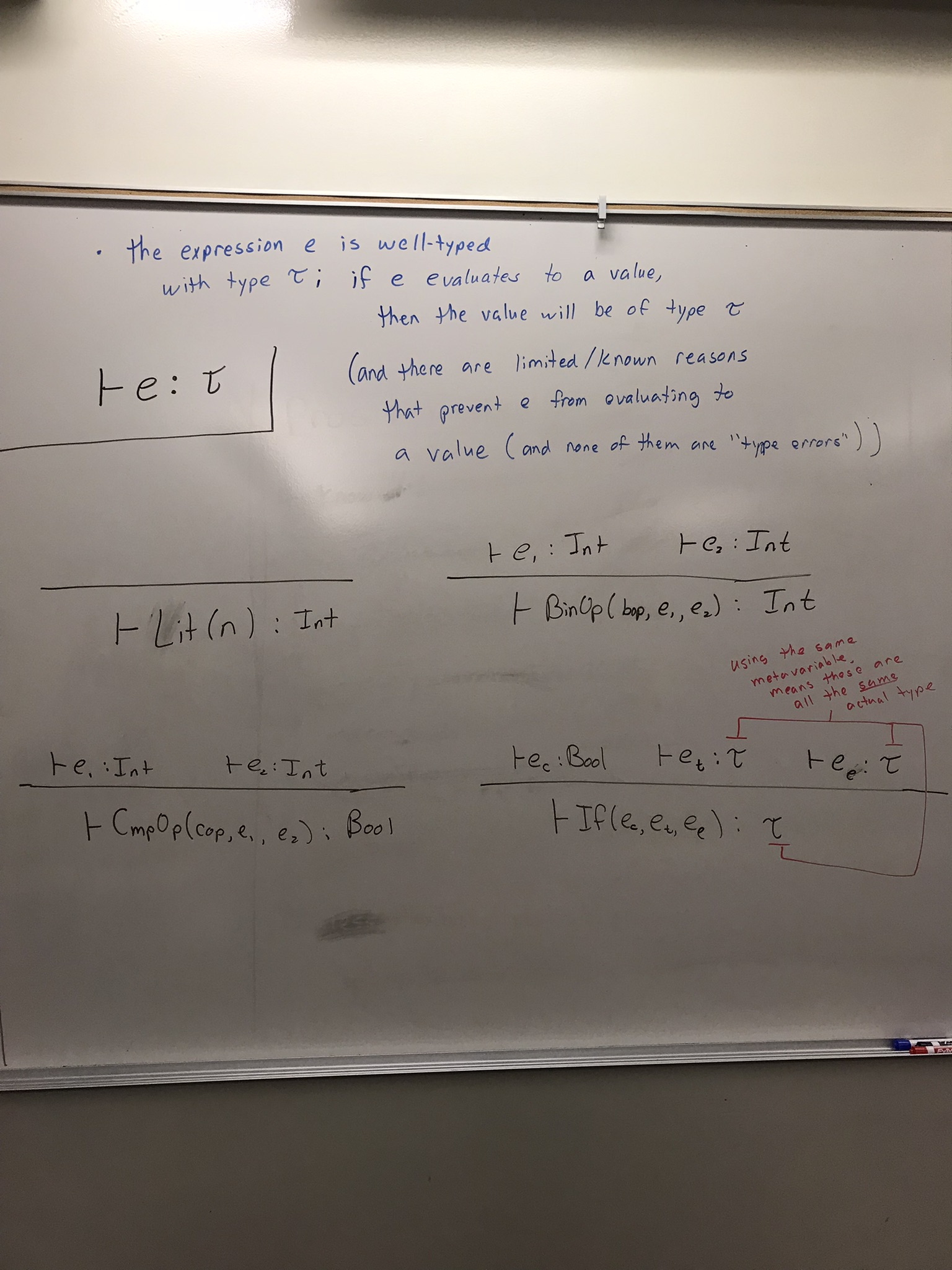
Mini Language
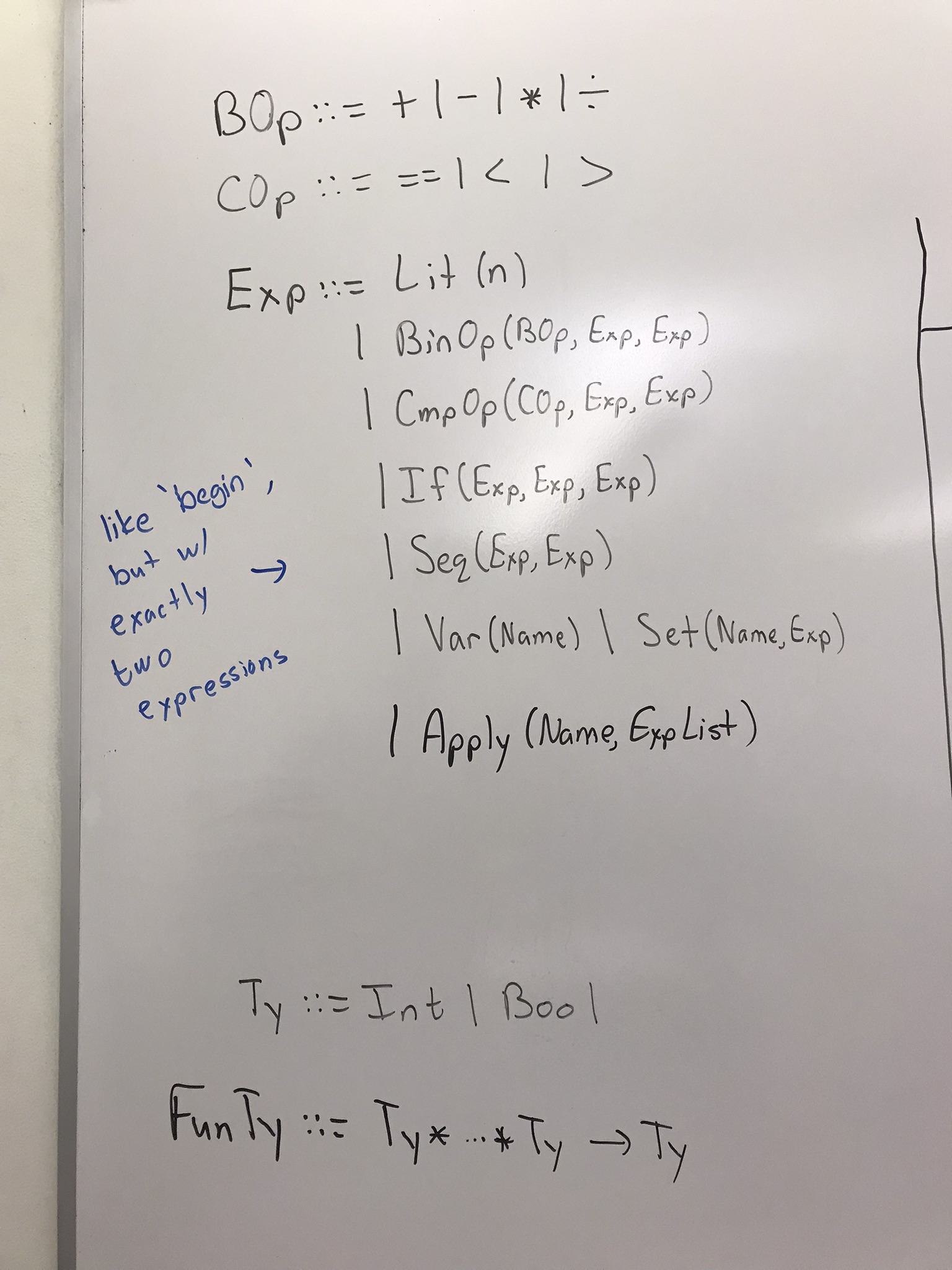
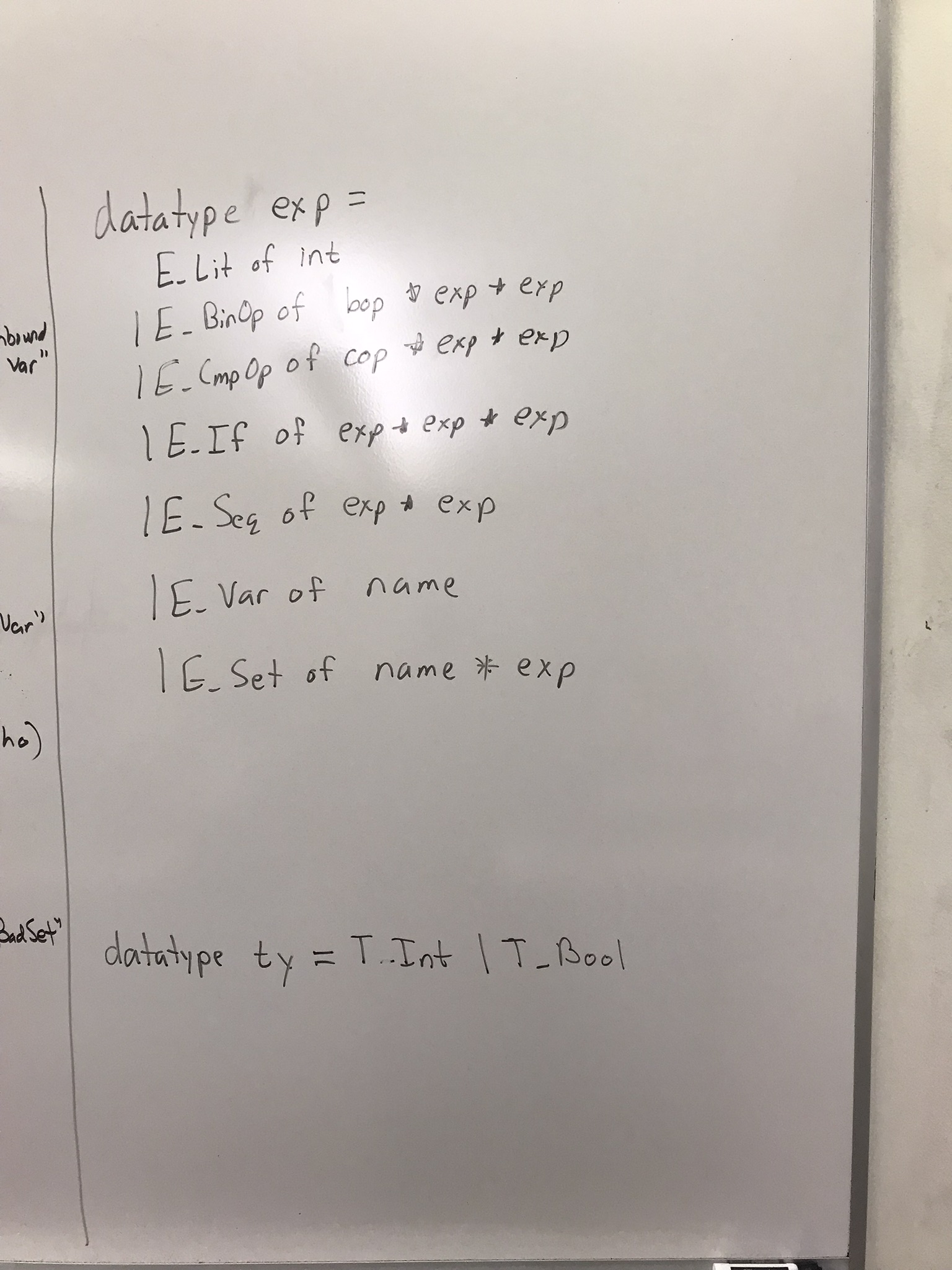
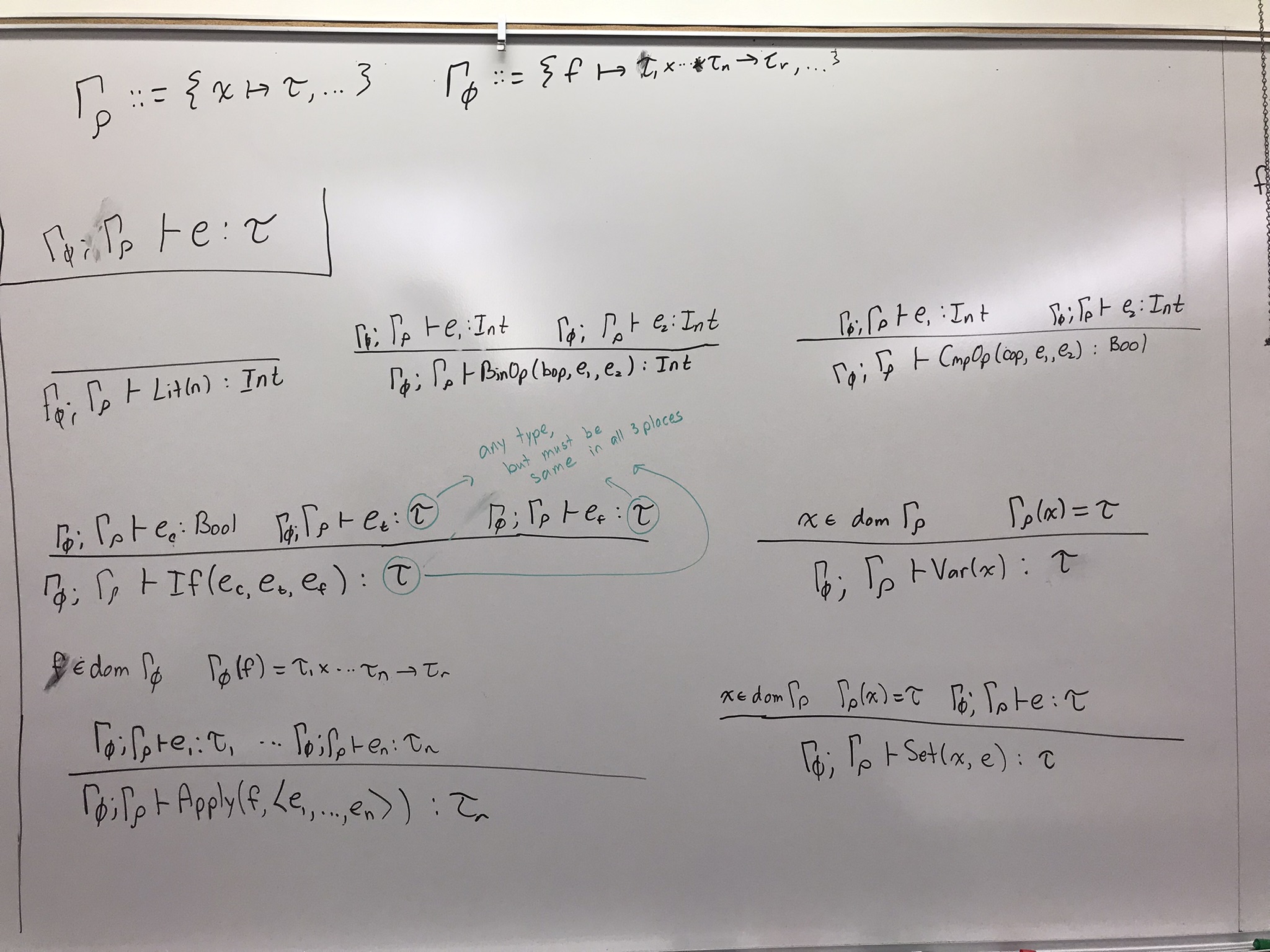
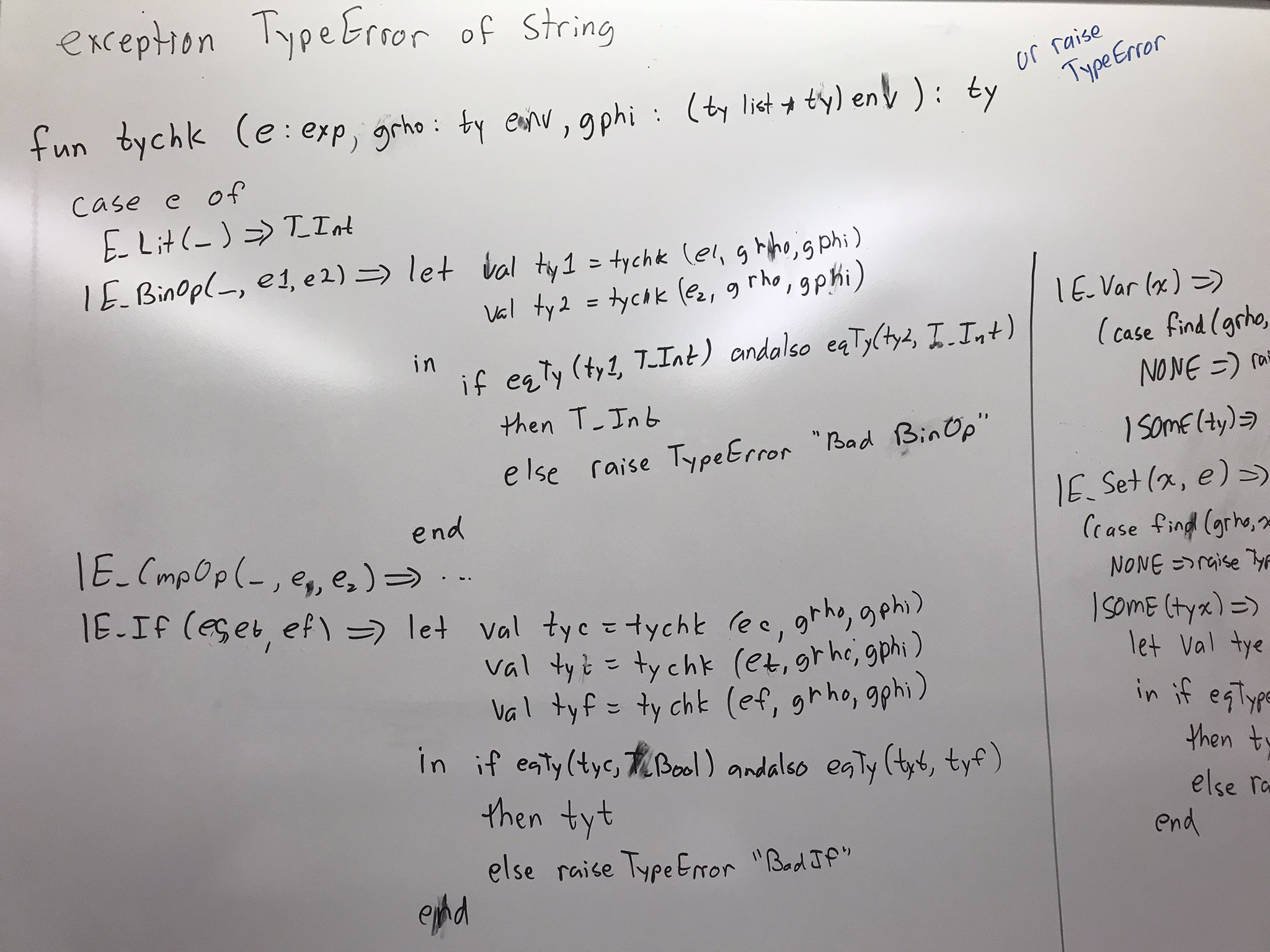
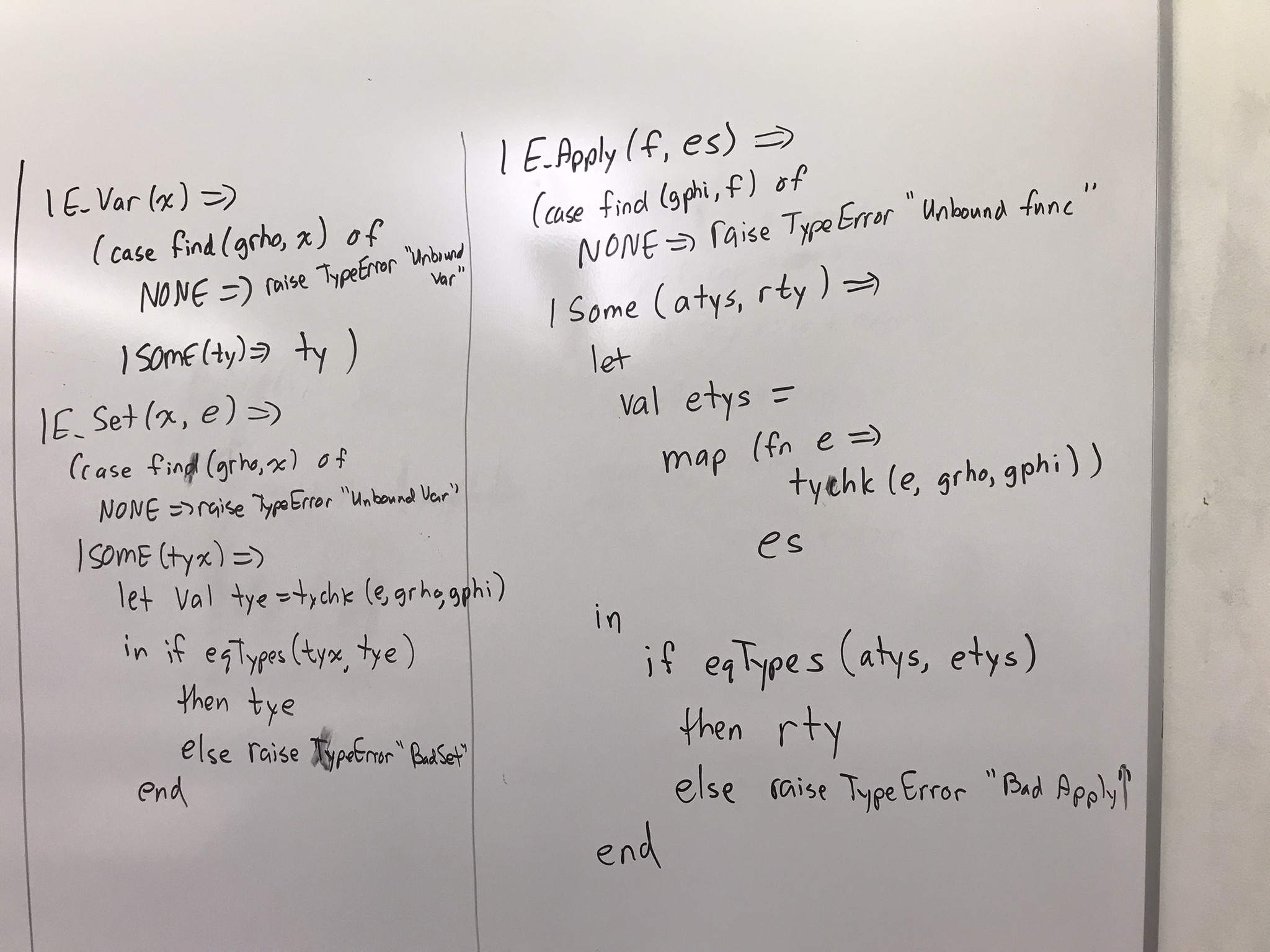